An Introduction to Javascript Objects
📣 Sponsor
Working with one dimensional arrays is great and all, but sometimes you need a little bit more than that. For storing more complex data structures in Javascript, we use objects. Objects allow us to have both a key and pair for our data.
How to make an object #
Making an object in Javascript is as easy as arrays. We use curly brackets to define our objects, and then have key/value pairs. A simple object looks like this:
let myObject = {
"someKey" : "someValue",
"someOtherKey" : "someOtherValue"
};
Objects give us context about our values, by allowing us to give them keys. Objects can be multi-dimensional, which just means they contain objects within themselves. They can also contain arrays. Therefore, the below is also a valid object:
let myObject = {
"someKey" : {
"subKey" : "aValue",
"anotherKey" : "anotherValue"
},
"someOtherKey": [ 1, 2, 3, 4, 5, 6 ],
"andFinally" : "oneMoreValue"
}
Object keys which are on the same level within an object need to be unique, so you can't have repeated keys.
Other ways to define objects
The above method is how we would most easily define an object, but you can also do it this way:
let myObject = new Object();
myObject.someKey = "someValue";
Above, we end up with an object with one key, called "someKey" with a value of "someValue".
Accessing Parts of an Object #
Since objects have keys, we can directly access them using either a dot (.) or square brackets([]). Let's take our first object as an example:
let myObject = {
"someKey" : {
"subKey" : "aValue",
"anotherKey" : "anotherValue"
},
"someOtherKey": [ 1, 2, 3, 4, 5, 6 ],
"andFinally" : "oneMoreValue"
}
// Both of these are the same way to access "someKey" in "myObject"
// As such, they both return "aValue"
let getMyKey = myObject.someKey;
let anotherWay = myObject["someKey"];
// If we want to get an object multiple layers deep, we can use multiple
// square brackets or dots:
let subKey = myObject.someKey.subKey;
let sameSubKey = myObject["someKey"]["subKey"];
You may notice that this format mimicks the format we use elsewhere, for instance document.querySelectorAll
. That is because document is an object which contains functions! You can actually find all the functions on objects like window
and document
by simply logging it in your console:
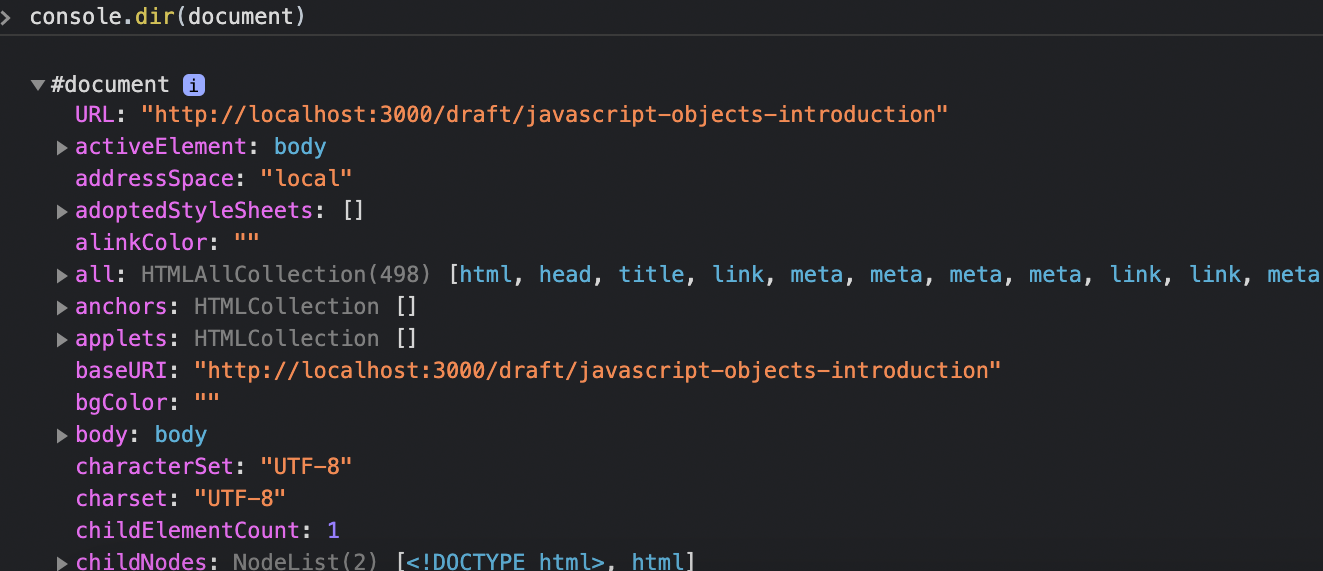
We can add functions to our object and call them as well:
let myObject = {
runFunction: function(name) {
console.log(`My name is ${name}`);
}
};
// Console logs "My name is John"
myObject.runFunction("John");
Javascript is often described as a prototype based language. Prototypes here refer to objects, and we call it that because Javascript hasn't historically used classes, and thus depended on objects like this.
Looping over Objects #
Since we have both keys and values with Javascript objects, accessing every item in an object becomes a little tricker. One of the easiest ways is to use a for loop:
let myObject = {
"someKey" : "someValue",
"someOtherKey" : "someOtherValue"
};
for(const prop in myObject) {
let key = prop; // "prop" here refers to the key name.
let value = myObject[prop] // We can now use "prop" to refer to get the value
}
Another way we can get all keys or values is to use Object.keys
and Object.values
. Both of these take an object, and covert either all of its keys or all of its values into an array.
Once we've got them in an array, we can use forEach on our arrays. For example:
let myObject = {
"someKey" : "someValue",
"someOtherKey" : "someOtherValue"
};
Object.keys(myObject).forEach(function(item) {
console.log(item); // This will console log both "someKey" and "someOtherKey"
let value = myObject[item]; // This will give us the value for both "someKey" and "someOtherKey"
});
let valueArray = Object.values(myObject); // This returns [ "someValue", "someOtherValue" ]
Using objects to create functional prototypes #
We've spoken about how Javascript is often described as a prototypical language. That means it is based around the structure of an object. In other articles we've spoken about how functions work.
Prototypes are essentially how Javascript functions what would be called classes in other languages. We use a base function and extend its prototype to give us functions relating to that function. In the below example:
- We have a function called Engine.
- Engine is an object, and thus it has some related functions. For our example we have one related function called go.
- An Engine can go, so we'll add the go function to our Engine's prototype.
- We then can run our go function, using variables defined in our Engine function, which we have defined with the this keyword.
- Since the variables are on the same object as the function go, they are accessible from within the go function.
Here is the code for our example:
let Engine = function(carName) {
this.horsePower = 100000;
this.speedMph = 10000000;
this.carName = carName;
}
Engine.prototype.go = function() {
console.log(`${this.carName} is going at ${this.speedMph}mph!`);
}
// This means we'll be able to more easily call our functions with Engine.go, rather than Engine.prototype.go
Engine.prototype.constructor = Engine;
let myCarEngine = new Engine('Marty McFly');
// This will console log 'Marty McFly is going at 10000000mph!'
myCarEngine.go();
Advanced Object Functions #
As with arrays, there are a number of complex ways to manipulate, access, and change objects. As such, we have made another article covering just that.
Click below to learn more about more advanced object functions.
More Tips and Tricks for Javascript
- Javascript Arrays - How to Remove Duplicate Elements
- Removing the last element of an array in Javascript
- Types may be coming to Javascript
- Javascript Temporal and How it Works
- How to Change CSS with Javascript
- How to get the last element of an Array in Javascript
- What are the three dots (...) or spread operator in Javascript?
- Inserting an Item into an Array at a Specific Index in Javascript
- Javascript Array Concat Method
- Javascript Operators and Expressions