An Introduction to Javascript
📣 Sponsor
Javascript is the versatile language which we use to build, develop, and add interactivity to the web. Javascript is one of the most widely used programming languages today, and it's used in a vast array of different circumstances. Javascript comes in two major forms:
- Client side, or frontend, which is included in HTML files, that adds interactivity to the website.
- Server side, or backend, for writing web servers, in the form of Node.JS.
Today, we'll be doing a quick introduction on what Javascript is, and what we use it for in web development. For this introduction, it is assumed you have an understanding of HTML and CSS.
What is Javascript?
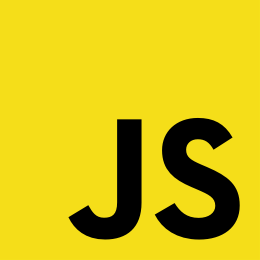
Javascript is a language based off the ECMA-262 standard. Javascript is a weakly typed language, meaning you don't have to define types when writing code in it. Javascript will dynamically interpret what type a variable might be, based on the context they are used in. As such, it is often said that Javascript has dynamic typing.
For example, in Javascript you may define a string (where a string is a sequence of characters), like so:
let myVariable = 'Some Sentence';
Javascript will automatically interpret this as having a type of String
. In other languages, though, you may have to explicitly mention the variable is a string, like this:
let myVariable:string = 'Some Sentence';
Javascript Support
Javascript is based off an ever evolving standard, as mentioned before. As such, new features are not always implemented immediately.
For example, Javascript comes built in to your browser (frontend), and different browsers (Chrome, Safari, Firefox, etc) have different support for new Javascript features. Therefore, some new Javascript features are not supported on all browsers. That means for brand new Javascript features, we have to check that it will work in a large variety of browsers before using it.
There are similar issues when installed on a server (backend), too. For example, Node.JS may not support all new Javascript features immediately.
What can I do with Javascript?
Let's briefly touch on some examples of what Javascript is used for. For the frontend, some examples of what Javascript is used for includes:
- Add or change CSS in an HTML document.
- Create new HTML elements programatically.
- Track what a user is doing, and react - i.e., do something when a user clicks.
- Change or delete HTML tags, i.e. change the classes an element has.
- Store data in objects and arrays, sometimes to be sent to the backend for storage.
On the backend, you can do things like:
- Create routes, and code what happens at certain URLs.
- Build servers that users can connect to.
- Build live APIs and Web Sockets that users can connect to.
- Handle compression of files for a faster experience.
- Manipulate data for storage in databases.
This is an incomplete list, but gives you an idea of the different kinds of things you'll be able to do with Javascript.
How do I add Javascript to Websites?
There are a few ways to include Javascript in a website:
- Inline scripts, that sit within HTML documents.
- In a separate file, included in HTML documents via the script tag.
- Within HTML elements, using attributes.
In an inline script
This is useful for Javascript you can't include easily in an external file, and requires the use of the <script>
tag. This can be inserted anywhere on your HTML web page, and looks like this:
<script type="text/javascript">
// Javascript goes here
</script>
In a separate file
If you have a separate file with the extension .js
, for example, script.js
, you can link to it via the <script>
tag too. For that, we use the src
attribute:
<script src="script.js"></script>
In a an HTML tag
You can also put Javascript directly in HTML tags. You may see this most frequently used in frameworks like React and Vue. For example, the onclick
attribute can contain Javascript. An example might look like this:
<button onclick="//javascript here"></button>
Using Javascript in Node.JS
We've spoken a lot about how Javascript can be included in HTML and via the <script>
tag. Typically, inserting Javascript into a website using those methods is for frontend work. If we want to work on the backend, we have to use Javascript differently. To make Javascript work on a computer directly, we typically use Node.JS, which can be installed via this link.
After it is installed, you can create standalone Javascript files, i.e. index.js
, and run them from the command line. That means we can run Javascript on a server or computer constantly. To run a Javascript file using this method you have to use terminal or command line. For example, if we had a file called index.js
, we would navigate to the folder your index.js
, and running the following command:
node index.js
What is Node.JS?
Node.JS is the backend version of Javascript. It's primarily used to do things like:
- Create routes, and code what happens at certain URLs.
- Build servers that users can connect to.
- Build live APIs and Web Sockets that users can connect to.
- Handle compression of files for a faster experience.
- Manipulate data for storage in databases.
Conclusion
Javascript is a flexible language used in many different places on the web. This introduction should give you an idea of what we use Javascript for, and how we create files and HTML tags to put Javascript in. It should also give you an idea of what type of language Javascript is. In the next part of our guide, we'll cover how Javascript variables work.
More Tips and Tricks for Javascript
- Scheduling and Runnning Recurring Cron Jobs in Node.JS
- Javascript Records and Tuples
- Javascript on Click Confetti Effect
- Checking if a value is a number in Javascript with isNaN()
- How Promises and Await work in Javascript
- Javascript Add Event Listener to Multiple Elements
- Creating 3d Animated Gradient Effects with Javascript and WebGL
- A Complete Guide to Javascript Maps
- Creating and Generating UUIDs with Javascript
- Using an Array as Function Parameter in JavaScript