How to Add Images to HTML Canvas
📣 Sponsor
When working with HTML Canvas, sometimes it's desirable to add images. In this article, let's look at how you can easily add images (like .jpeg
and .png
) to your HTML canvas.
If you're brand new to HTML Canvas, start with our "Getting Started with HTML Canvas" guide.
How to add Images to HTML Canvas
Adding images to HTML canvas depends upon the Image()
constructor, which lets us interact with images in Javascript. For this guide, I'll be using an image from Pexels by figenkokol.
To start, create your HTML canvas element as you normally would:
<canvas id="canvas" width="300" height="300"></canvas>
Now, let's look at the HTML. We first create a new Image()
, and then set its url (i.e. src
) to the image we want to show:
let canvas = document.getElementById('canvas');
let ctx = canvas.getContext('2d');
// Create our image
let newImage = new Image();
newImage.src = 'https://fjolt.com/images/misc/202203281.png'
// When it loads
newImage.onload = () => {
// Draw the image onto the context
ctx.drawImage(newImage, 0, 0, 250, 208);
}
When the image loads (newImage.onload
), then we draw the image onto our canvas. To do that, we use ctx.drawImage()
. The syntax is shown below.
ctx.drawImage(image, x, y, width, height)
If declared like this, ctx.drawImage() only has 5 arguments:
image
- the image we want to use, generated from ournew Image()
constructor.x
- the x position on the canvas for the top left corner of the image.y
- the y position on the canvas for the top left corner of the image.width
- the width of the image. If left blank, the original image width is used.height
- the height of the image. If left blank, the original image height is used.
The above code will produce the following canvas:
Now we've successfully drawn an image onto an HTML canvas element, using just Javascript.
Cropping Images in HTML Canvas
Using ctx.drawImage
function, we can also crop images. This version of the function accepts a slightly different syntax, but lets us crop an image as we see fit.
ctx.drawImage(image, cx, cy, sw, sh, x, y, width, height)
If declared like this, ctx.drawImage() has 9 arguments:
image
- the image we want to use, generated from ournew Image()
constructor.cx
- this is how far from the top left we want to crop the image by. So if it is 50, the image will be cropped 50 pixels from the left hand side.cy
- this is how far from the top we want to crop the image by. So if it is 50, the image will be cropped 50 pixels from the top side.sw
- this is how big we want the image to be from the point ofcx
. So if 100, the image will continue for 100px fromcx
, and then be cropped at that point.sh
- this is how big we want the image to be from the point ofch
. So if 100, the image will continue for 100px fromch
, and then be cropped at that point.x
- the x position on the canvas for the top left corner of the image.y
- the y position on the canvas for the top left corner of the image.width
- the width of the image. If left blank, the original image width is used.height
- the height of the image. If left blank, the original image height is used.
If you prefer the visual, here is how cropping works with this method:
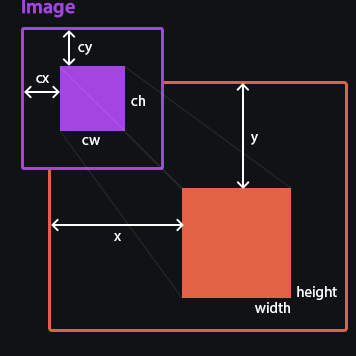
Let's look at an example. Nothing really changes, except for the syntax of ctx.drawImage
.
let canvas = document.getElementById('canvas');
let ctx = canvas.getContext('2d');
// Create our image
let newImage = new Image();
newImage.src = 'https://fjolt.com/images/misc/202203281.png'
// When it loads
newImage.onload = () => {
// Draw the image onto the context with cropping
ctx2.drawImage(newImage, 20, 20, 500, 500, 0, 0, 250, 208);
}
Note: the cropping effect will use the original image size - so if your image is 1000px wide, as this one is, we have to crop it according to those dimensions. We can then use x, y, width, height
to draw the image onto any size we like.
The above, will produce the following canvas:
More Tips and Tricks for HTML
- How to save HTML Canvas as an Image
- Creating Shapes with HTML Canvas
- Getting Started with HTML Canvas
- HTML tags for text
- Creating Dark Mode, for Lazy People
- How to Draw Text with HTML Canvas
- SEO HTML Meta Tag Reference List
- How to Add Images to HTML Canvas
- Everything you need to know about HTML Input Types
- How to wrap text in HTML Canvas