How to use v-for in Vue
📣 Sponsor
Often times when we are creating application, the data we use determines what we show to the user. For example, in a to-do application, we may have multiple to-do list items. In Vue, it is easy to display multiple data points through the v-for
attribute in our Vue templates.
How to use v-for in Vue
Let's suppose we have some data we are storing on a single page component. Our .vue
document looks a bit like this:
<div id="locations">
</div>
<script>
export default {
data() {
return {
locations: [
{ name: 'London', date: '11/02/2022', numberOfPeople: 4, complete: true },
{ name: 'Paris', date: '12/01/2022', numberOfPeople: 2, complete: true },
{ name: 'Tokyo', date: '04/06/2021', numberOfPeople: 6, complete: true },
{ name: 'Mumbai', date: '08/10/2021', numberOfPeople: 10, complete: true },
{ name: 'New York', date: '12/12/2022', numberOfPeople: 14, complete: true },
{ name: 'Dubai', date: '10/02/2023', numberOfPeople: 12, complete: false },
{ name: 'Shanghai', date: '04/02/2020', numberOfPeople: 2, complete: true }
]
}
}
}
</script>
Our intention is to display all of our "location" data in our template. We are using static data here - but v-for
is reactive, just like all of Vue. So if an API updates this data, it'll feed through to our template.
In this example, using v-for
is a no brainer. All we have to do is update or <template>
tag to loop through each item. Let's take a look at how we'd do that:
<div id="locations">
<div class="location-item" v-for="(item, index) in locations" :key="index">
<p>We travelled to on with people.</p>
</div>
</div>
After updating our code, we should have something that looks like this:
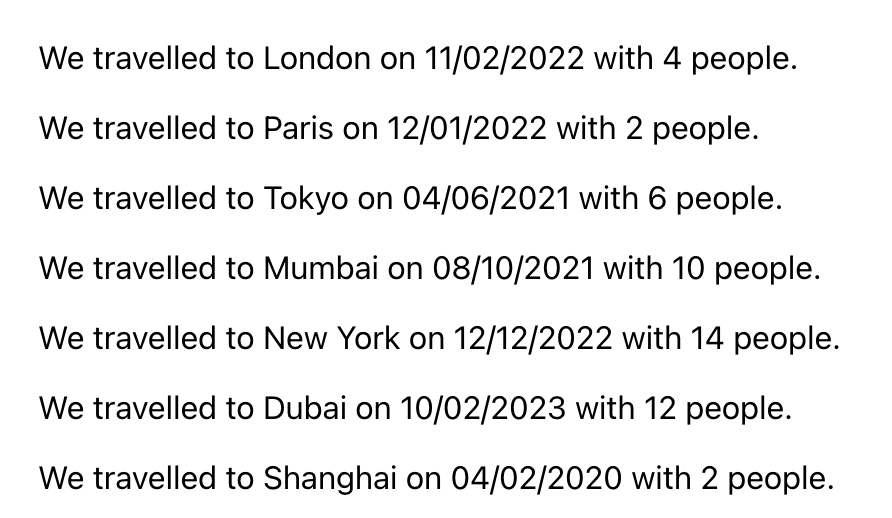
So now all of our data is easily displayed in paragraph form. Our .location-item
div fully contains the logic for our v-for
loop:
<div class="location-item" v-for="(n, i) in locations" :key="i">
When we say (item, index) in locations
, item refers to one item in our loop - so we can call item.name
to get London, or Mumbai from our data set. index
refers to the index of that element.
You might also notice we wrote :key="index"
. Every v-for
loop item requires a key. For this example, we are using the index as our key. If you leave this out - you will get an error in Vue.
How to use v-if and v-for together in Vue
In Vue, we can't use v-for
and v-if
together, as they often conflict. In our data above, we have a field called completed
, which is true if the journey is over, and false if it hasn't happened yet. If we wanted to only show completed journeys, we need to add our v-if
to a child HTML element. If we add it to the element with v-for
, it won't work!
As such, we could hide any element where completed is false by adding changing our template to look like this:
<div id="locations">
<div class="location-item" v-for="(item, index) in locations" :key="index">
<p v-if="item.completed === true">We travelled to on with people.</p>
</div>
</div>
Nested v-for loops in Vue
It's worth also mentioning that nested v-for
loops are possible, and follow the same pattern as we've covered in this article. Here is an example of a set of nested v-for
loops, where we loop through a list of countries, and their states:
<div id="countries">
<div class="country-item" v-for="(item, index) in countries" :key="index">
<h2> States:</h2>
<p v-for="(state, i) in item.states" :key="i">state.name</p>
</div>
</div>
<script>
export default {
data() {
return {
countries: [
{
name: "UK",
states: [{
name: "London",
lowerCaseName: "london"
},
{
name: "Scotland",
lowerCaseName: "scotland"
}]
// More...
},
{
name: "India",
states: [{
name: "Madhya Pradesh",
lowerCaseName: "madhya-pradesh"
}]
// More...
}
]
}
}
}
</script>
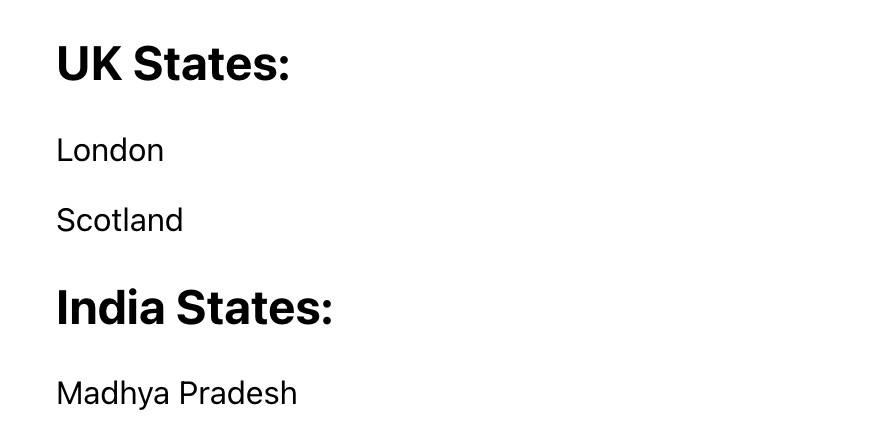
Conclusion
That's all for v-for
loops. We've looked at:
- How to use v-for loops on
data()
. - How to use nested v-for loops.
- Combining v-for and v-if.
Vue is super fun to use once you start. You can find more Vue content here.
More Tips and Tricks for Vue
- The Difference Between the Composition API and Options API in Vue
- A Guide to Understanding Vue Lifecycle Hooks
- Vue Tips: Dynamic CSS with Vue Reactive Variables
- Navigation between views in Vue with Vue Router
- How to give Props Default Values in Vue
- A Guide to Events in Vue
- How to Watch for Nested Changes in Vue
- Creating your first Vue App
- Vue Tips: Optimize your Vue Apps with v-once and v-memo
- How to use Watchers in Vue